Modelos de Formulario¶
Puedes usar modelos de Pydantic para declarar campos de formulario en FastAPI.
Información
Para usar formularios, primero instala python-multipart
.
Asegúrate de crear un entorno virtual, activarlo, y luego instalarlo, por ejemplo:
$ pip install python-multipart
Nota
Esto es compatible desde la versión 0.113.0
de FastAPI. 🤓
Modelos de Pydantic para Formularios¶
Solo necesitas declarar un modelo de Pydantic con los campos que quieres recibir como campos de formulario, y luego declarar el parámetro como Form
:
from typing import Annotated
from fastapi import FastAPI, Form
from pydantic import BaseModel
app = FastAPI()
class FormData(BaseModel):
username: str
password: str
@app.post("/login/")
async def login(data: Annotated[FormData, Form()]):
return data
🤓 Other versions and variants
from fastapi import FastAPI, Form
from pydantic import BaseModel
from typing_extensions import Annotated
app = FastAPI()
class FormData(BaseModel):
username: str
password: str
@app.post("/login/")
async def login(data: Annotated[FormData, Form()]):
return data
Tip
Prefer to use the Annotated
version if possible.
from fastapi import FastAPI, Form
from pydantic import BaseModel
app = FastAPI()
class FormData(BaseModel):
username: str
password: str
@app.post("/login/")
async def login(data: FormData = Form()):
return data
FastAPI extraerá los datos de cada campo de los form data en el request y te dará el modelo de Pydantic que definiste.
Revisa la Documentación¶
Puedes verificarlo en la interfaz de documentación en /docs
:
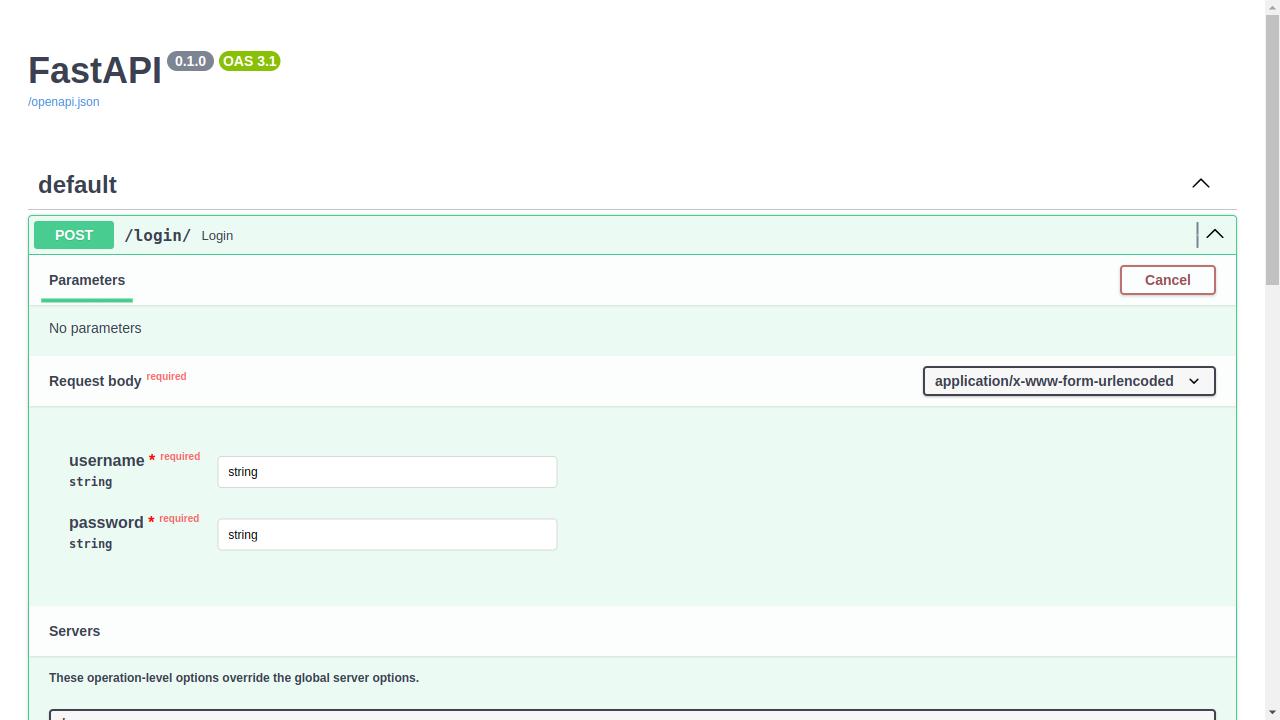
Prohibir Campos de Formulario Extra¶
En algunos casos de uso especiales (probablemente no muy comunes), podrías querer restringir los campos de formulario a solo aquellos declarados en el modelo de Pydantic. Y prohibir cualquier campo extra.
Nota
Esto es compatible desde la versión 0.114.0
de FastAPI. 🤓
Puedes usar la configuración del modelo de Pydantic para forbid
cualquier campo extra
:
from typing import Annotated
from fastapi import FastAPI, Form
from pydantic import BaseModel
app = FastAPI()
class FormData(BaseModel):
username: str
password: str
model_config = {"extra": "forbid"}
@app.post("/login/")
async def login(data: Annotated[FormData, Form()]):
return data
🤓 Other versions and variants
from fastapi import FastAPI, Form
from pydantic import BaseModel
from typing_extensions import Annotated
app = FastAPI()
class FormData(BaseModel):
username: str
password: str
model_config = {"extra": "forbid"}
@app.post("/login/")
async def login(data: Annotated[FormData, Form()]):
return data
Tip
Prefer to use the Annotated
version if possible.
from fastapi import FastAPI, Form
from pydantic import BaseModel
app = FastAPI()
class FormData(BaseModel):
username: str
password: str
model_config = {"extra": "forbid"}
@app.post("/login/")
async def login(data: FormData = Form()):
return data
Si un cliente intenta enviar datos extra, recibirá un response de error.
Por ejemplo, si el cliente intenta enviar los campos de formulario:
username
:Rick
password
:Portal Gun
extra
:Mr. Poopybutthole
Recibirá un response de error indicando que el campo extra
no está permitido:
{
"detail": [
{
"type": "extra_forbidden",
"loc": ["body", "extra"],
"msg": "Extra inputs are not permitted",
"input": "Mr. Poopybutthole"
}
]
}
Resumen¶
Puedes usar modelos de Pydantic para declarar campos de formulario en FastAPI. 😎